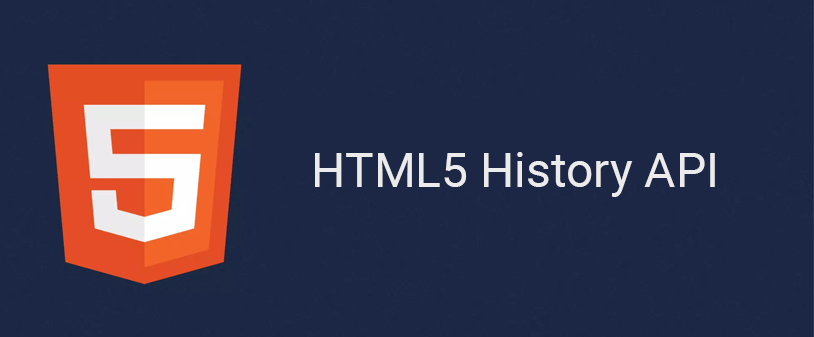
How to change url without refreshing the page?
The basic idea behind project I worked on is
- When the popup opens, the url changes without reloading the page and the share button is containing this url.
- Once the popup is closed, the url changes to the original url.
- Open relevant popup on page load when you click on a link containing this url.
I decided to modify the url by adding a url parameter telling JavaScript which popup to open.
For this post I use https://www.josefzacek.cz/blog/how-to-change-url-without-refreshing-the-page as domain name and I will modify url to https://www.josefzacek.cz/blog/how-to-change-url-without-refreshing-the-page?popup=parameter
Just click the buttons below to see it in action
Click me to pass "WordPress" parameter to url
Click me to pass "JavaScript" parameter to url
Click me to pass "HTML" parameter to url
Reset
Code for example above:
<style>
.click-me-btn {
text-decoration: underline;
cursor: pointer
}
</style>
<span class="click-me-btn" id="WordPress">Click me to pass "WordPress" parameter to url</span>
<span class="click-me-btn" id="JavaScript">Click me to pass "JavaScript" parameter to url</span>
<span class="click-me-btn" id="HTML">Click me to pass "HTML" parameter to url</span>
<span class="click-me-btn" id="reset">Reset</span>
<script>
var originUrl = window.location.href;
function change_url(state, title, url) {
window.history.pushState(state, title, url);
}
jQuery(".click-me-btn").click(function(){
var buttonId = jQuery(this).attr("id");
if(buttonId == "reset"){
change_url("state", "title", originUrl.split('?')[0]);
} else {
change_url("state", "title", "?popup=" + buttonId );
}
})
</script>
pushState() function
When pushState()
is called, the new state is pushed onto the history stack, and the URL in the address bar is updated to the specified URL. However, it does not trigger a page refresh or navigate to a new page. This allows you to update the URL and handle the state change using JavaScript, enabling you to provide a smooth user experience within a single page.
Here’s a breakdown of the parameters:
state (optional): This parameter represents an object that contains data associated with the new state. It allows you to store custom information related to the state being added. You can pass null
if you don’t have any state data to store.
title (optional): This parameter represents the title of the new state. Browsers generally ignore this parameter, so it is often set to an empty string or a short description of the state.
url (optional): This parameter represents the new URL that will be displayed in the browser’s address bar. The URL should be relative to the current page’s URL. If you want to update only the URL without affecting the page’s content, you can pass null
.
Get url when page load:
var originUrl = window.location.href;
Function to change url:
function change_url(state, title, url) {
window.history.pushState(state, title, url);
}
When user clicks on span.click-me-btn get button id.
If button has id='”reset”, reset the url otherwise change it as required
jQuery(".click-me-btn").click(function(){
var buttonId = jQuery(this).attr("id");
if(buttonId == "reset"){
change_url("state", "title", originUrl.split('?')[0]);
} else {
change_url("state", "title", "?popup=" + buttonId );
}
})