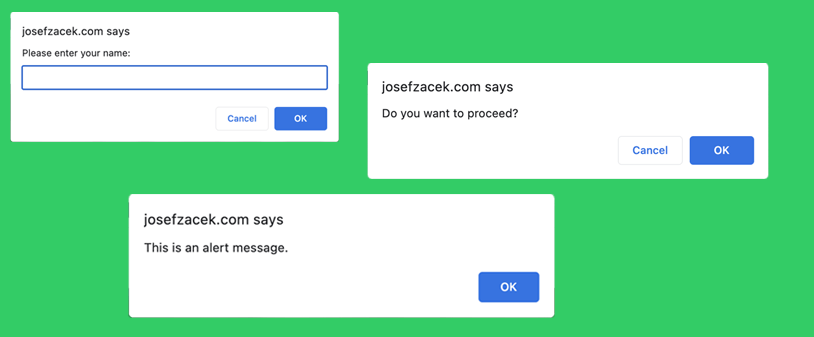
What's the difference between alert, confirm and prompt?
Alert, confirm, and prompt are JavaScript functions that allow you to interact with users through dialog boxes in web applications. They are used to display messages, gather user input, and confirm actions.
Here’s a breakdown of their differences:
alert dialog box
The alert function displays a simple dialog box with a message and an “OK” button. It is often used to provide information or notifications to the user.
The user cannot interact with the page or perform any actions until they dismiss the alert by clicking the “OK” button.
It only has one button option and cannot gather any input from the user.
<script>
alert("This is an alert message.");
</script>
confirm dialog box
The confirm function displays a dialog box with a message and two buttons: “OK” and “Cancel.”
It is commonly used to ask the user for confirmation before proceeding with a certain action. The user can choose to either confirm or cancel the action.
The function returns a Boolean value (true if “OK” is clicked, and false if “Cancel” is clicked).
<script>
var result = confirm("Do you want to proceed?");
if (result) {
// User clicked "OK"
} else {
// User clicked "Cancel"
}
</script>
prompt dialog box
The prompt function displays a dialog box with a message, an input field for the user to enter text, and two buttons: “OK” and “Cancel.” It is used to gather input from the user.
The function takes two arguments: the first is the message to display, and the second (optional) is the default value to be displayed in the input field.
The function returns the text entered by the user, or null if the user clicked “Cancel.”
<script>
var userInput = prompt("Please enter your name:");
if (userInput !== null) {
// User clicked "OK" and entered a value
alert("Hello, " + userInput + "!");
} else {
// User clicked "Cancel"
}
</script>
Note: Alert is added to the example above to show user input
Summary
alert is for displaying information.
confirm is for getting simple user confirmation.
prompt is for getting user input through a text field.
Keep in mind that these functions provide basic interaction and are primarily used for simple user interactions in web applications.